Very large image manipulation
What if an image size is much larger than the amount of RAM available?
- Load and save images of hundreds of MPix
- Lean memory usage for any sized image
- Both bitmaps and vector images
- Incredible performance
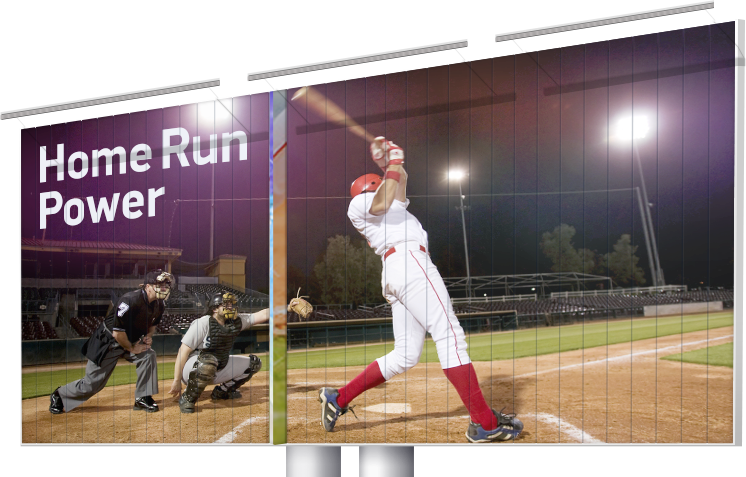
Processing large images without OutOfMemoryException
The traditional way to process an image is to allocate a buffer large enough to hold all its pixels and decode an image file into memory.
It works great for regular photos, but what if the image dimensions are really large? For example, satellite images? Billboard designs? Panoramic images stitched out of dozens of photos?
The obvious solution is to read an image partially, process one piece of the image, save that part of the image, and then proceed.
Instead of loading an image into memory, Graphics Mill allows you to construct a "pipeline" (graph) of effects and "push" the image through this pipeline. It will get a small piece of input.jpg, rotate it, resize and write it to output.jpg. Then it will proceed with the next chunk of input.jpg until it reaches the end of a file.
Large vector images are also supported
This smart "pipeline" technology is used not only with bitmaps. This approach also works with vector images like PDF or SVG. Graphics Mill easily processes them even if they are really large.
Memory friendly does not mean complicated
You may be worried that this chunk-by-chunk processing approach is too difficult and requires writing more code. It really isn't! With a convenient and intuitive API, all the powerful image processing functions of Graphics Mill are seamless and completely automatic.
Moreover, you don't always have to use pipelines. It is absolutely fine to combine both classic and pipeline approaches as best suits your needs.. Code examples and documentation for each feature is available for both classic and pipeline APIs.
Let's compare the classic and pipeline APIs:
Classic API
using (var bitmap = new Bitmap("In.jpg")) { bitmap.Transforms.Resize(320, 240); bitmap.Save("Out.jpg"); }
Memory-friendly pipeline API
using (var reader = ImageReader.Create("In.jpg")) using (var resize = new Resize(320, 240)) using (var writer = ImageWriter.Create("Out.jpg")) { Pipeline.Run(reader + resize + writer); }
Orders of magnitude faster than other SDKs
When processing thousands of images simultaneously, performance is crucial. We work hard to keep Graphics Mill faster than other well-known imaging SDKs.
Operation | Graphics Mill | System.Drawing | WIC |
---|---|---|---|
Resize 10000x10000 to 2000x2000 * | 20 ms | 199 ms | 105 ms |
Load 1000x1000 JPEG * | 2 ms | 23 ms | 6 ms |
Get 100x100 thumbnail from JPEG 1000x1000 * | 5 ms | 50 ms | 30 ms |
*Speeds may vary on your servers due to differences in hardware
We take the algorithms' performance seriously. For example, one of our regression test suites is created especially to ensure that changes in Graphics Mill does not slow it down.
Robust image processing
Graphics Mill is robust enough to handle extremely large image loads. Just feed it with any number of images and Graphics Mill will do a perfect job without defects or failures.